Next.js Version 13, introduced by Vercel during the Next.js conference in October 2022, comes brimming with an array of fresh features.
The overarching objective of this release is to enhance the development process by making it swifter, more straightforward, and higher in performance. This is achieved through code splitting, caching, and resource-loading optimizations. As a result, your Next.js application will exhibit quicker load times and execution, ultimately delivering an improved user experience.
While we've previously explored the pros and cons of Next.js, it's now time to delve into what Version 13 brings to the forefront. In this article, we'll go through the new features and enhancements, encompassing elements such as the App Router, data fetching, rendering, Turbopack, and an array of novel hooks, including specialized functions.
Additionally, with the upcoming release of Next.js 14 on October 26 this year, we'll also tackle the latest version. Let's get started!
The App Router
Version 13 of Next.js introduced a fresh App Router, constructed upon React Server Components. This innovative router offers support for shared layouts, nested routing, loading states, error handling, and a host of other capabilities.
The App Router works in a new directory named {app}. Working in harmony with the page's directory to enable incremental adoption, the Router provides the ability to selectively transition certain routes of your application to the new behavior while retaining the existing behavior for other routes in the page's directory.
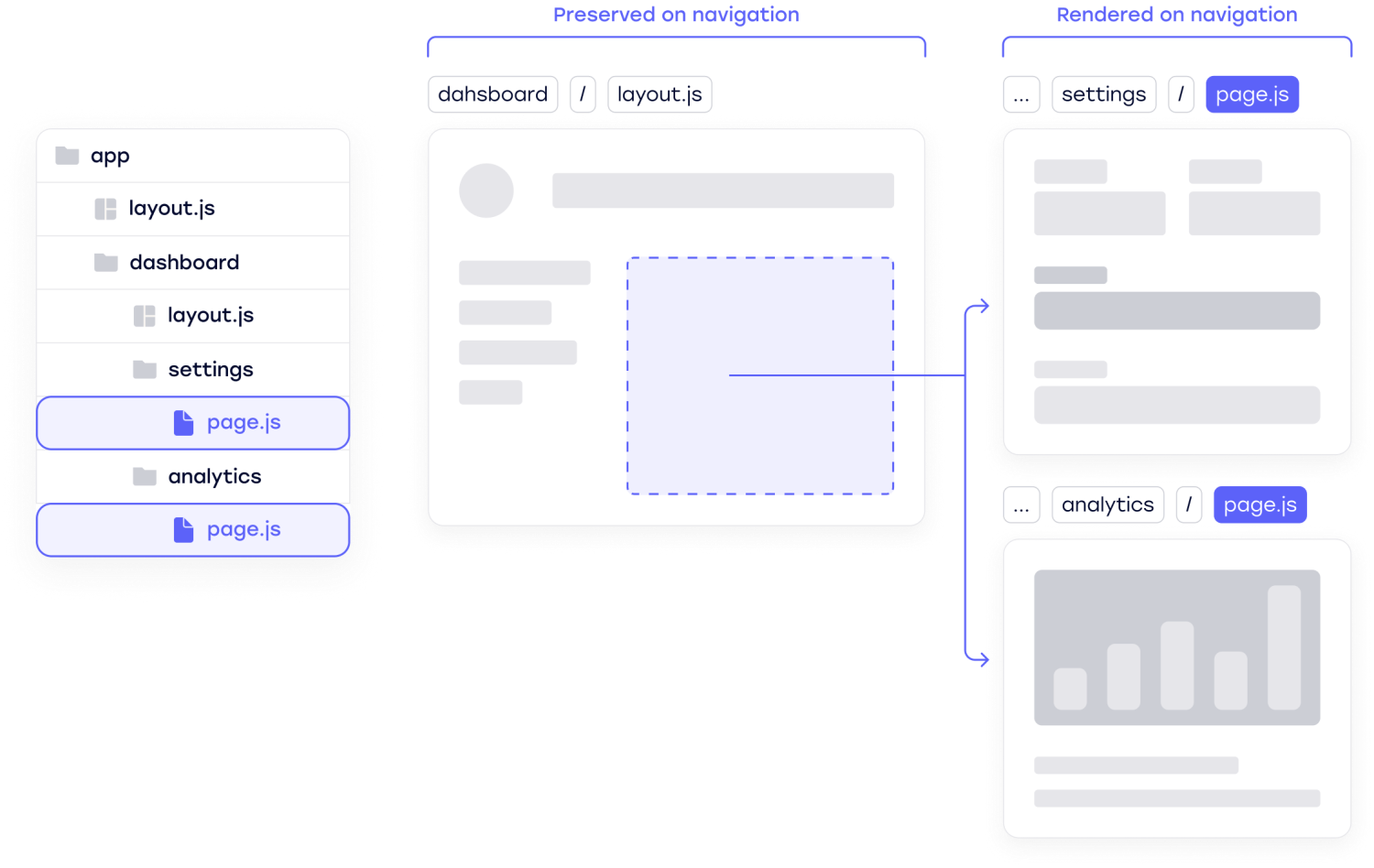
For new applications, Next.js suggests using the App Router. Furthermore, if you have an existing application, you can slowly start using the App Router. You also have the option to use both routers in the same application.
It's important to note that the App Router takes precedence over the Pages Router. Routes in different directories should not lead to the same URL path, as this would trigger a build-time error to avoid any conflicts.
To learn more about App Router and Routing Fundamentals with Next.js, follow the links to the official Next.js website.
Is Data Fetching More Convenient?
Yes! Next.js 13 introduces simplified options for data fetching. First, the new ways of doing things are closely connected to React Server Components. In Next.js 13, all components are now {server} by default. We can use the familiar async/await way of doing things in React components. Here is an example of how it can be done:
1async function getData() {
2 const res = await fetch('YOUR_API_ENDPOINT_LINK');
3
4 if (!res.ok) {
5 throw new Error('Failed to fetch data');
6 }
7
8 return res.json();
9}
10
11export default async function Page() {
12 const data = await getData();
13
14 return <main />;
15}
This change makes it a lot simpler and more adaptable when it comes to getting data. The great thing is that you can spread out the part of your code that gets data from the server into different places and keep it close to the parts of your app that actually use that data.
In Next.js 13, when you want to access the same data in various components within a tree, like the current user, the framework conveniently caches similar fetch requests in a temporary cache. This clever feature eliminates the problem of prop drilling, so now, when you fetch the same resource during a render pass, it sends just one HTTP request, saving you from extra costs. You can now fetch data where you need it without any concerns.
What Are Server Actions?
Next.js 13 brings a new feature called Server Actions, offering a powerful way to run server code without the need for API endpoints.
With Server Actions, manual API endpoint creation becomes unnecessary. Instead, you define asynchronous server functions that can be directly called from your components. These Server Actions seamlessly integrate with Next.js caching and revalidation, updating cached data and cache keys when a form is submitted.
With traditional applications like PHP, a route can have a single {get} and {post} request, but with the introduction of Server Actions in Next.js you can have as many "endpoints" as you wish with each route. This gives you the best of both worlds, as you will be having the benefits of traditional apps and the innovations that Next.js and React introduced. Server Actions also eliminate the need for the browser to refresh when a form is submitted. In just one network roundtrip, Next.js can provide both the updated UI and refreshed data.
Additionally, Server Actions offer the flexibility to invalidate the Next.js Cache on demand. You can invalidate an entire route segment using {revalidatePath} or a specific data fetch with a cache tag using {revalidateTag}.
1'use server'
2
3import { revalidatePath } from 'next/cache'
4
5export default async function submit() {
6 await submitForm()
7 revalidatePath('/')
8}
9'use server'
10
11import { revalidateTag } from 'next/cache'
12
13export default async function submit() {
14 await addPost()
15 revalidateTag('posts')
16}
Let’s Check the Rendering in Next.js 13!
The first important rendering change is the introduction of the previously mentioned App Router alongside the Pages Router. When developing our app, we can opt for either of these routers.
This adjustment has an impact on how we utilize components in Next.js. We now have two distinct React component types: Server Components and Client Components.
Both types are server-rendered, but the crucial difference lies in Server Components being exclusively “server only”. This means no additional JavaScript is transmitted to the client for hydration, resulting in quicker page loading and less bandwidth usage.
NOTE: Components within the App Router are automatically classified as Server Components.
Server-side rendering (SSR) enhances a web page's initial load time by crafting the page's starting state on the server and delivering it as a fully rendered HTML document to the browser. This not only boosts SEO friendliness but also accelerates page rendering by relieving the browser from executing JavaScript for rendering.
In typical JavaScript-based websites (such as SPAs) the website is usually put together and shown in the browser while you use it. Next.js improves upon this by compiling and rendering the website at build time by default.
This means it generates a set of ready-to-go files, including HTML, JavaScript, and CSS, just like SSR. As a result, users don't have to sit around for a lot of JavaScript to load and run in their browser before they can see the website. It's faster and more efficient.
Turbopack Acceleration
Turbopack is a bundler designed to accelerate your Next.js app development. It is an incremental bundler optimized for JavaScript and TypeScript, written in Rust.
You can speed up local work with {next dev --turbo} and, in the near future, boost production builds with {next build --turbo}.
In Next.js, you can utilize Turbopack in both the pages and app directories to enhance local development speed. To activate Turbopack, simply use the {--turbo} flag when starting the Next.js development server.
Here are the key points to keep in mind when using Turbopack:
Incremental Efficiency: Turbopack is designed to be incremental, avoiding redundant work. Once it handles a task, it won't repeat it.
Ecosystem Versatility: It offers comprehensive support for various technologies like TypeScript, JSX, CSS, CSS Modules, WebAssembly, and more, straight out of the box.
Rapid Hot Module Replacement: Regardless of your app's size, Turbopack ensures fast HMR for swift updates during development.
React Server Components: Turbopack seamlessly integrates with React Server Components for native support.
Multiple Environment Targets: You can build and optimize for multiple environments simultaneously, including Browser, Server, Edge, SSR, and React Server Components.
Powering Production Builds: Turbopack is set to enhance Next.js production builds, both locally and in the cloud, for efficiency and speed.
Hooks & Functions
One thing that is worth mentioning is that TypeScript is the default when creating a new project.
Moreover, this version introduces many new hooks and functions, including:
{useRouter} for programmatic redirects.
{useSearchParams} for reading URL Search Parameters.
{useSelectedLayoutSegment} for fetching the current route segment and {useSelectedLayoutSegments} for accessing all child pages within the current layout segment.
{usePathname} for retrieving the current pathname.
Furthermore, the special functions for Server Components that this version brings are:
{cookies} to read cookies on the server side.
{fetch} for making server-side requests, extending the web fetch API.
{headers} for reading HTTP headers on the server.
{notFound} to dynamically return a 404 response from the server.
{redirect} for server-side redirection.
The Future of Next.js: Version 14
Next.js 13 introduces a range of new optimizations that significantly enhance various processes, including Server Side Rendering. It's important to note that Version 13 addressed certain shortcomings from the previous version (regarding splitting, caching, and resource loading) and that it continues to push for innovation, fostering ongoing progress.
All these improvements result in better SEO and faster page load times. Coupled with Turbopack and the new hooks and functions, Next.js becomes even more powerful.
Yet, new opportunities are on the horizon with Next.js 14. On October 26, 2023, we were introduced to fresh improvements and news in this latest version, which encompasses:
5,000 Turbopack’s passed tests for the App & Pages Router. When tested on vercel.com with a sizable Next.js application, the results showed remarkable improvements, including up to 53.3% faster local server startup and up to 94.7% faster code updates with Fast Refresh. Now that 90% of tests for {next dev} are passing, you can expect consistently faster and more reliable performance when using {next dev --turbo}.
Server Actions have made it into a stable release, allowing you to securely perform server-side actions with just a function defined in your React component. Here's an example from Medium.com.
1export default function Page() {
2 async function create(formData: FormData) {
3 'use server';
4
5 const id = await createItem(formData);
6 }
7 return (
8 <form action={create}>
9 <input type="text" name="name" />
10 <button type="submit">Submit</button>
11 </form>
12 );
13}
Partial Prerendering is now in preview, potentially resolving the debate between SSR and SSG. It combines a rapid initial static response with dynamic content streaming based on your React Suspense boundaries. This approach provides the performance benefits of static sites along with the dynamism of server-rendered apps, offering the best of both worlds.
Metadata options such as {viewport}, {colorScheme}, and {themeColor} have been removed from metadata. With the release of Next.js 14, new options, {viewport} and {generateViewport}, have been introduced to replace these existing options, while all other metadata options remain unchanged.
The Next.js 14 also offers a learning opportunity via Next.js Learn for everyone keen to learn more about these new updates. If you're interested in leveraging Next.js for your business, you’re in the right place here at FatCat. Don't hesitate to get in touch and discover how our expertise and services can benefit you.
We’re looking forward to following the future of this framework and sharing the latest trends with you. Stay tuned for updates!
Share this article: